The Python ecosystem
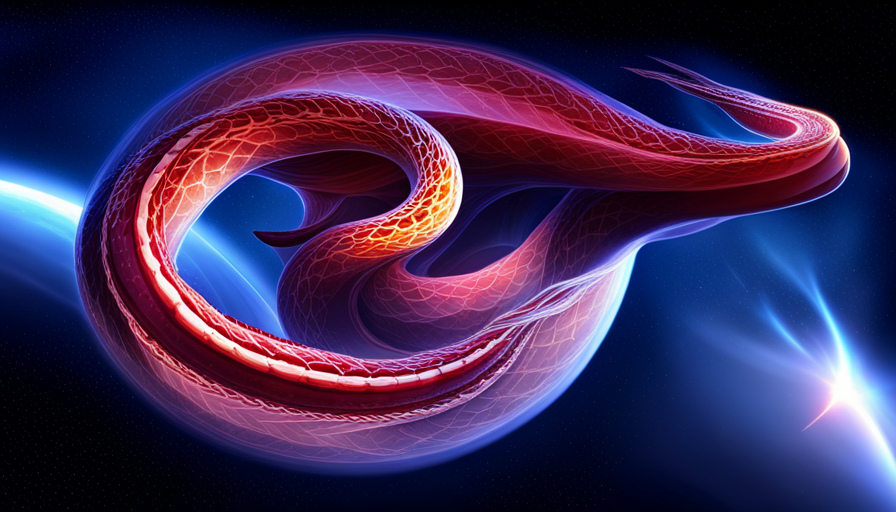
Introduction
Python has been a faithful companion throughout my programming journey.
But let's be honest, there have been times when Python's terminology has left me scratching my head. Many projects share very similar names such as PyPI, PyPy and mypy and it can be confusing especially for newcomers.
In this post, I want to provide an overview of the main projects from the Python ecosystem. By the end of it you should be able to differentiate CPython from Cython!
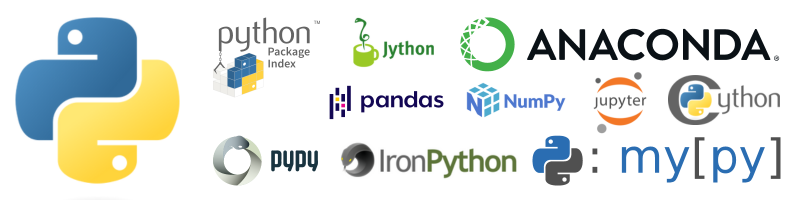
The Python language
Let's start with the basics.
Python itself is an interpreted, high-level, general-purpose programming language that emphasizes code readability and simplicity. It has a clean and easy-to-understand syntax, making it an excellent choice for beginners as well as experienced programmers.
Python is known for its extensive standard library, which provides a vast array of modules and functions for various tasks, including file manipulation, network programming and web development.
def fib(n):
a, b = 0, 1
while a < n:
print(a, end=' ')
a, b = b, a+b
print()
Python interpreters
But Python itself is just a language. In order to write software in Python, you need an interpreter.
CPython
CPython is the default implementation of the Python programming language. It is written in C and is often referred to as the "reference implementation". CPython compiles Python code into bytecode and then executes it on a virtual machine.
It is the most widely used implementation and is considered the de facto standard for Python. Whenever Python is upgraded with new features, language developers upgrade the CPython version as well, to the extent that both words can almost be used interchangeably.
PyPy
PyPy is an alternative implementation of Python that aims to improve performance.
It includes a just-in-time (JIT) compiler that dynamically translates Python bytecode into machine code, optimizing execution speed. PyPy often outperforms CPython for workloads that involve long and computationally intensive tasks.
IronPython
IronPython is an implementation of Python that runs on the .NET framework.
It allows developers to write Python code that can interact with other .NET languages and libraries. IronPython is particularly valuable for integrating Python with existing .NET projects and leveraging the capabilities of the .NET ecosystem.
Jython
Jython is an implementation of Python that runs on the Java Virtual Machine (JVM).
It allows developers to write Python code that can interact with Java code and libraries. Jython provides access to the vast Java ecosystem, enabling developers to leverage existing Java frameworks and libraries in their Python projects.
Python packages
PyPI (Python Package Index)
PyPI, also known as the Python Package Index, is a repository of software packages for Python.
It hosts a vast collection of libraries and modules that extend Python's capabilities. Developers can use these packages to add functionalities to their Python projects without reinventing the wheel.
pip
pip is a package manager for Python that simplifies the process of installing and managing Python packages from PyPI.
It is a command-line tool. For example, the following command will install the library Pandas from PyPI:
pip install pandas
Tools
mypy
mypy is a static type-checking command-line tool for Python.
It allows developers to annotate their code with type hints and performs static analysis to detect potential type errors. By adding type annotations, developers can catch bugs early and improve code maintainability. mypy is widely adopted in the Python community and is recommended for large-scale projects.
# fibonacci.py
from typing import Iterator
def fib(n: int) -> Iterator[int]:
a, b = 0, 1
while a < n:
yield a
a, b = b, a + b
mypy fibonacci.py
>>> Success: no issues found in 1 source file
Cython
Cython is a programming language and a superset of Python.
It allows developers to write Python code that can be compiled to C or C++ for better performance. By combining Python's ease of use with the speed of C, Cython is an excellent choice for performance-critical applications.
def primes(int nb_primes):
cdef int n, i, len_p
cdef int[1000] p
if nb_primes > 1000:
nb_primes = 1000
len_p = 0 # The current number of elements in p.
n = 2
while len_p < nb_primes:
# Is n prime?
for i in p[:len_p]:
if n % i == 0:
break
# If no break occurred in the loop, we have a prime.
else:
p[len_p] = n
len_p += 1
n += 1
# Let's copy the result into a Python list:
result_as_list = [prime for prime in p[:len_p]]
return result_as_list
Python for data science
Jupyter
Jupyter is a web-based interactive computing environment widely used by data scientists and researchers. It supports the creation and sharing of documents containing live code, equations, visualizations, and narrative text. Jupyter notebooks allow users to write and execute Python code in an interactive and exploratory manner, making it an invaluable tool for data analysis, machine learning, and scientific research.
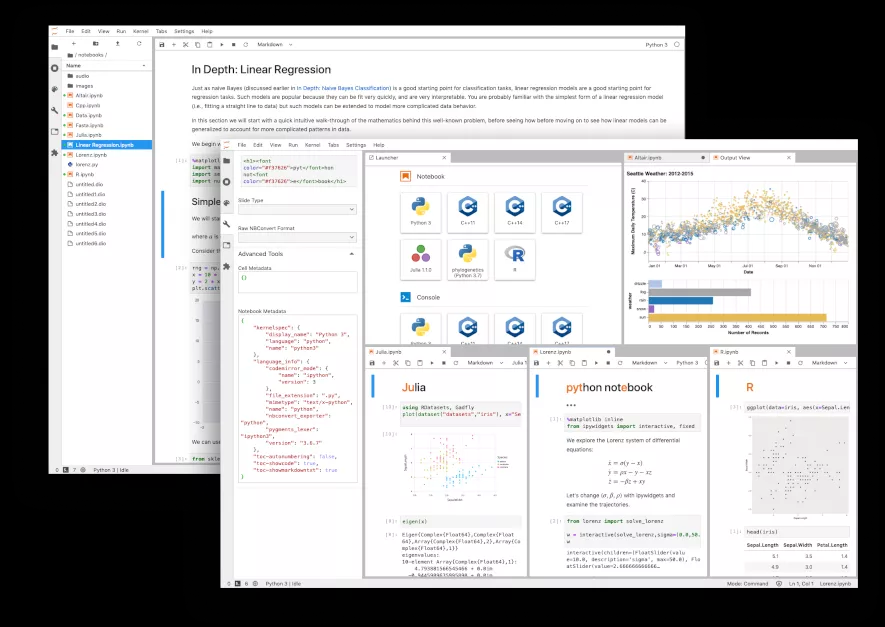
Anaconda and Miniconda
Anaconda and Miniconda are two popular package management systems and distribution platforms for Python and other programming languages that are widely used for data science and scientific research.
Anaconda is a full-featured distribution that includes a comprehensive collection of pre-built packages, tools, and libraries for scientific computing, data analysis, and machine learning. Anaconda also provides the Anaconda Navigator, a graphical user interface (GUI) that allows users to manage packages, environments, and projects easily.
Miniconda is a lightweight version of Anaconda that includes only the essential components: Python interpreter, conda package manager, and a few core packages. It offers a minimal and flexible base for building custom environments.
Conclusion
Python stands out as a highly versatile programming language with a thriving ecosystem of projects and tools. The availability of different implementations such as CPython or PyPy, along with the convenience of package management through pip or conda, allows empowers developers to choose the options for their projects.
Additionally, tools like mypy and Cython contribute to code quality and performance optimization, while Jupyter provide specialized capabilities for interactive data exploration.
Hopefully it is a little bit clearer for you now!